Ping Result Array Manager Failed
I know what you are thinking, another ping script? Yes, there are thousands of ping tools and scripts out there and every IT administrator has a couple handy that they use on a regular basis. I ended up writing this one because I could not find PowerShell code that met the specific criteria that I needed. I was working with one of my peers on SCCM client health. In large enterprise environments it is an ongoing challenge to ensure that all devices on the network are accounted for and managed. We wrote some PowerShell to query some databases and come up with a list of machines we knew about that were not managed by SCCM. The next question I had was how many were actually on the network.
- This will result in 'ORA-12545: Connect failed because target host or object does not exist' Maybe there was an entry for myserver in the local hosts file, but it specified a bad IP address.
- Re: the compatibility, I just wanted to confirm the basics. I think you are on the right track. I had similar issues during my 5.1 setup. I suggest you look at the 'Advanced Settings' of your remote site.
I wanted my ping code to meet the following criteria.
- Fast, so pings asynchronously
- Reliable, can recover and continue if errors are encountered
- Uses .NET or PS Cmdlets, I don’t want to have to externally launch anything
- Returns nicely formatted data
- Is modular, I want to be able to re-use this code in other scripts easily
The examples I found online had most of these features, but not all. Once I started writing it I thought this might be useful to the community so I added the txt file as an input and csv file as an output as my original code was just a few functions I called. Note, this does require PowerShell 3.0 or higher.
Syntax
.Ping.ps1 –InputFilePath c:tempnames.txt –MaxConcurrent 100 –TimesToPing 4 –TimeoutInSeconds 90 –ResolveNames true
I am ignoring Test-Connection:AsJob as it failed with a Quota Violation in the final two sets of results. So the results look rather interesting. The smaller batches show that using Test-Connection with the –AsJob parameter performed right along with the SendPingAsync approaches.
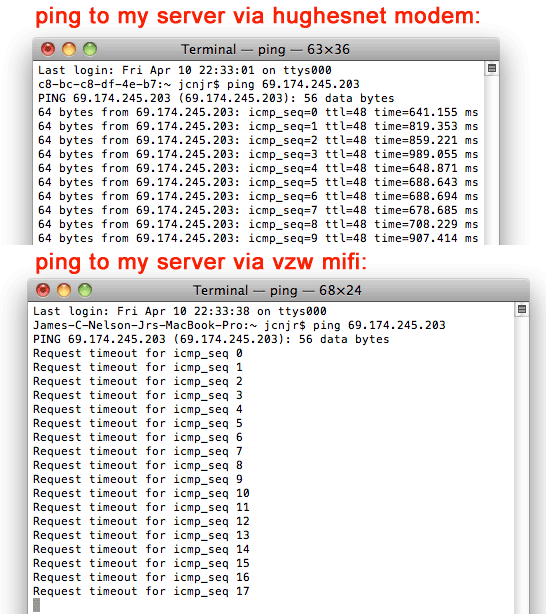
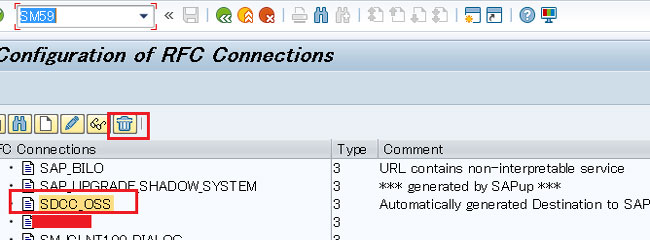
Parameters
Name | Description |
InputFilePath | Text file with a list of names or IP addresses you want to ping. Each name/IP should be on its own line in the file. |
MaxConcurrent | How many jobs / threads you want to use for pinging. |
TimesToPing | How many times you want to ping each name/IP. |
TimeoutInSeconds | Optional: If some jobs / threads get stuck running, this will ensure that we recover and continue if the timeout is reached. This timeout only applies to Test-Connection (Ping) and the GetHostEntry (DNS) parts of the script. If this is not specified it defaults to 120 seconds. |
ResolveNames | Optional: Set to true if you want to resolve the names using DNS. |
Output
Part 1: This should happen very quickly
Sending Ping Command to 500 Machines… (Uses the Test-Connection Cmdlet with –AsJob)
Getting Ping Results……… (Gets the results from the Cmdlet. The timeout passed in applies to this part of the script. If you see a + here than one of the ping jobs failed and was resubmitted.)
Received Ping Results From 500 Machines
Part 2: This should happen quickly. Note, you can grab more properties from the ping objects in this function if you want. I did notice that getting certain properties cause the function to slow down significantly such as IPv4Address and IPv6Address which are of type System.Net.IPAddress.
Formatting Ping Results…… (Creates an array of objects for the ping results)
Formatted Ping Results for 500 Machines
Part 3: This can take a while
Resolving DNS Names for 500 Machines...*..*…… (GetHostEntry .NET call. The * means MaxConcurrent was hit, which in the case of DNS resolution is hardcoded to 5 since that seemed to work best in my testing.)
Getting DNS Results… (Gets the results of GetHostEntry. The timeout passed is applies to this part of the script. If you see a + here than one of the DNS resolution jobs failed and was resubmitted.)
Received DNS Results From 500 Machines
Formatting DNS Results.. (Adds the DNS information to the array of objects returned from Part 2)
Formatted DNS Results for 500 Machines
Part 4: This should happen quickly, just outputting the results
---Statistics---
Total Time Elapsed: 02 Minutes and 36 Seconds
Total Names Pinged: 500
Hosts that Responded: 250
DNS Names Resolved: 350
Percentage of Hosts that Responded at Least Once: 50%
CSV Output Location: C:tempPingResults.csv
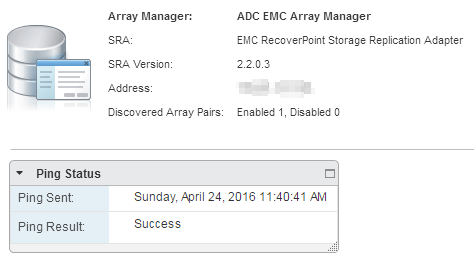
Testing
I tested this on two machines using my home internet connection (Verizon FIOS) against 500 internet addresses. If you don’t resolve DNS names then this script finishes very quickly on a fast machine and even faster if you choose to only do 1 ping instead of the normal 4. You can increase the MaxConcurrent setting to get faster results from the ping but if you start seeing a lot of “+” signs in the output then then the jobs are failing (although we should recover from this). Unfortunately because of limitations in the Test-Connection Cmdlet I have to do the DNS resolution and pings separately and the DNS resolution is the longest part of the script. You can use the Win32_PingStatus class directly to do both at the same time, but I’m not sure which method is faster.
Ping Result Array Manager Failed Windows 10
ResolveNames = true, MaxConcurrent=100, TimesToPing=4
HP 8570W (i7/32GB), WS2012, Gigabit Ethernet: Completed in 1 minute, 15 seconds.
Microsoft Surface Pro (i5/4GB), Windows8, Gigabit Ethernet: Completed in 2 minutes, 36 seconds.
Ping Result Array Manager Failed Download
ResolveNames = false, MaxConcurrent=100, TimesToPing=4
HP 8570W (i7/32GB), WS2012, Gigabit Ethernet: Completed in 19 seconds.
Microsoft Surface Pro (i5/4GB), Windows8, Gigabit Ethernet: Completed in 33 seconds.
Code